Golang如何处理Json数据?-几个技巧和实例
Golang如何处理Json数据?- 几个技巧和实例
在现代编程中,Json已经成为了一种通用的标准数据格式,很多应用都在使用Json进行数据传送和存储。Golang是一种非常优秀的编程语言,其内置了对Json的强大支持,使得Golang开发人员能够非常方便地处理Json数据。本文将介绍一些Golang处理Json数据的技巧和实例。
1. 解析Json数据
对于Golang开发人员而言,最常用的Json处理就是解析Json数据。Golang内置了encoding/json包,该包提供了Unmarshal()函数,可以将Json数据解析为Golang的结构体或map对象。
例如,我们有如下的Json数据:
`json
{
"name": "张三",
"age": 18,
"score":
}
我们可以将其解析为一个struct对象:`gotype Student struct { Name string json:"name" Age int json:"age" Score int json:"score"}func main() { jsonStr := { "name": "张三", "age": 18, "score": } var student Student json.Unmarshal(byte(jsonStr), &student) fmt.Printf("姓名:%s,年龄:%d,成绩:%v", student.Name, student.Age, student.Score)}
输出结果为:
姓名:张三,年龄:18,成绩:
2. 生成Json数据
Golang内置的encoding/json包也可以将Golang结构体或map对象转换为Json数据。使用json.Marshal()函数可以将Golang对象转换为Json字符串,使用json.MarshalIndent()函数可以将Golang对象转换为格式化的Json字符串。
例如,我们有如下的结构体:
`go
type Student struct {
Name string json:"name"
Age int json:"age"
Score int json:"score"
}
我们可以将其转换为Json字符串:`gostudent := Student{Name: "张三", Age: 18, Score: int{95, 85, 90}}jsonBytes, _ := json.Marshal(student)jsonStr := string(jsonBytes)fmt.Println(jsonStr)
输出结果为:
{"name":"张三","age":18,"score":}
使用json.MarshalIndent()函数将生成格式化的Json字符串:
`go
student := Student{Name: "张三", Age: 18, Score: int{95, 85, 90}}
jsonBytes, _ := json.MarshalIndent(student, "", " ")
jsonStr := string(jsonBytes)
fmt.Println(jsonStr)
输出结果为:
{
"name": "张三",
"age": 18,
"score":
}
3. 解析Json数组Json数组是一种非常常见的数据格式,Golang内置的encoding/json包也提供了对Json数组的支持。对于Json数组,我们可以将其解析为Golang内置的slice或数组类型。例如,我们有如下的Json数组:`json
我们可以将其解析为Golang的slice类型:
`go
jsonStr := ""
var scores int
json.Unmarshal(byte(jsonStr), &scores)
fmt.Printf("%v", scores)
输出结果为:
4. 处理Json中的Null值Json中的Null值表示一个空值,Golang内置的encoding/json包也对Null值进行了支持。当我们将Json数据解析为Golang对象时,如果Json中某个属性的值为Null,那么对应的Golang属性将被赋值为零值。例如,我们有如下的Json数据:`json{ "name": "张三", "age": null, "score": }
我们可以将其解析为如下的结构体:
`go
type Student struct {
Name string json:"name"
Age int json:"age"
Score int json:"score"
}
对于Json中的Null值,我们可以通过指定Golang属性的类型为*int、*float64等指针类型,使得该属性在Json中对应Null值时,被解析为nil。例如,我们有如下的Json数据:`json{ "name": "张三", "age": null}
我们可以将其解析为如下的结构体:
`go
type Student struct {
Name string json:"name"
Age *int json:"age"
}
这样,当Json中的age属性为Null时,Golang中的Age属性将被赋值为nil。5. 自定义Json解析和生成Golang内置的encoding/json包提供了很多方便的函数来解析和生成Json数据,但有时我们需要一些更加复杂且个性化的Json处理方式。这时,我们可以使用encoding/json包中的Unmarshaler和Marshaler接口来自定义Json解析和生成。例如,我们有如下的Json数据:`json{ "timestamp": 1637988314, "data": { "name": "张三", "age": 18, "score": }}
我们想要将其解析为如下的结构体:
`go
type Student struct {
Name string json:"name"
Age int json:"age"
Score int json:"score"
}
type Data struct {
Timestamp int64 `json:"timestamp"
Student *Student json:"data"
}
func (d *Data) UnmarshalJSON(data byte) error {
type Alias Data
aux := &struct {
*Alias
Student json.RawMessage json:"data"
}{
Alias: (*Alias)(d),
}
if err := json.Unmarshal(data, aux); err != nil {
return err
}
if aux.Student != nil {
s := new(Student)
if err := json.Unmarshal(aux.Student, s); err != nil {
return err
}
d.Student = s
}
return nil
}
func main() {
jsonStr := {
"timestamp": 1637988314,
"data": {
"name": "张三",
"age": 18,
"score":
}
}`
var data Data
json.Unmarshal(byte(jsonStr), &data)
fmt.Printf("时间戳:%d,姓名:%s,年龄:%d,成绩:%v", data.Timestamp, data.Student.Name, data.Student.Age, data.Student.Score)
}
在上面的代码中,我们使用Unmarshaler接口实现了自定义的Json解析逻辑。首先,我们定义了一个Data结构体,该结构体包含了时间戳和一个指向Student结构体的指针。然后,我们实现了UnmarshalJSON()函数,并对Data结构体进行了扩展。在UnmarshalJSON()函数中,我们首先定义了一个辅助结构体Alias,用于保存Data结构体的属性;然后,在解析Json数据时,我们将Json对象解析为aux结构体;最后,我们对Student属性进行了单独解析,避免将其直接解析为Json对象。6. 处理Json中的特殊字符在Json数据中,有一些特殊字符(如双引号、分号等)会影响Json的解析和生成。为了避免这种情况出现,我们可以使用encoding/json包中的EscapeHTML()函数来转义特殊字符。例如,我们有如下的结构体:`gotype Student struct { Name string json:"name" Desc string json:"desc"}
其中,Desc属性可能包含有特殊字符,我们可以将其转义为Json字符串:
`go
student := Student{Name: "张三", Desc: ""}
studentBytes, _ := json.Marshal(student)
jsonStr := string(studentBytes)
jsonStr = strings.Replace(jsonStr, "\u0026", "\\u0026", -1)
jsonStr = strings.Replace(jsonStr, "\u003c", "\\u003c", -1)
jsonStr = strings.Replace(jsonStr, "\u003e", "\\u003e", -1)
jsonStr = strings.Replace(jsonStr, "\u0027", "\\u0027", -1)
fmt.Println(jsonStr)
输出结果为:
{"name":"张三","desc":"\u003cscript\u003ealert(\u0027hello\u0027)\u003c/script\u003e"}
在上面的代码中,我们使用了strings.Replace()函数将特殊字符进行了转义。
总结
Golang内置的encoding/json包为Golang开发人员处理Json数据提供了非常方便的支持。本文介绍了一些Golang处理Json数据的技巧和实例,包括如何解析Json数据、生成Json数据、处理Json中的Null值、自定义Json解析和生成以及处理Json中的特殊字符。希望本文能够对Golang开发人员处理Json数据提供帮助。

猜你喜欢LIKE
相关推荐HOT
更多>>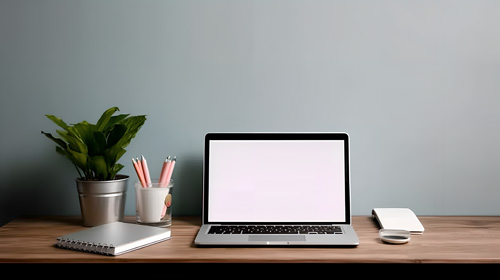
Goland中的GoModules管理你的依赖关系
Goland中的Go Modules:管理你的依赖关系为了更好地管理Go项目的依赖关系,Go Modules是一种新的解决方案,它已经成为了Go开发人员的标准选择。...详情>>
2023-12-21 21:24:37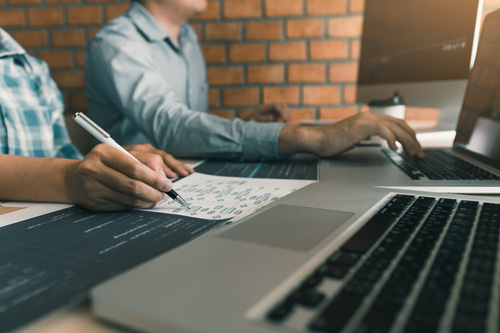
GolangvsPython哪个更适合开发微服务?
随着微服务的流行,越来越多的开发者开始关注哪种编程语言最适合构建微服务。在这方面,Golang和Python是两种非常流行的语言。那么,哪一个更适...详情>>
2023-12-21 16:36:36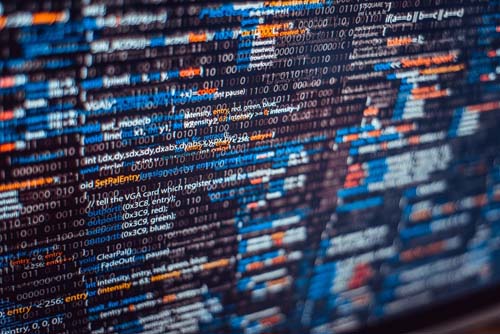
Golang中的Web框架对比GinvsBeego
Golang中的Web框架对比:Gin vs BeegoGolang语言自发布以来在互联网领域颇受欢迎,尤其是在Web开发中的应用越来越广泛,而Gin和Beego是两个比较...详情>>
2023-12-21 14:12:36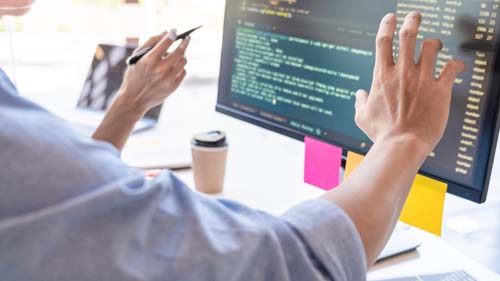
如何利用云计算技术来优化企业级金融分析和风险控制?
随着金融行业的不断发展,企业级金融分析和风险控制越来越重要。而云计算技术也不断发展,为企业级金融分析和风险控制提供了更加优化的解决方案...详情>>
2023-12-21 04:36:36热门推荐
goland常用快捷键详解,让你的开发速度飞起来!
沸基于Goland的高质量Go语言程序开发实践分享!
热Goland中的GoModules管理你的依赖关系
热GoLand技巧如何在代码中使用GoModules
新GoLand中的代码格式化让你的代码更加整洁和一致
Golang如何处理Json数据?-几个技巧和实例
GolangvsPython哪个更适合开发微服务?
GolangWeb框架性能对比GinvsBeego
Golang中的Web框架对比GinvsBeego
goland插件推荐-提升Go开发效率,从这里开始
Linux操作系统为什么成为互联网基础设施的首选?
为什么你应该考虑使用容器编排工具来管理你的应用程序
什么是OpenStack?为什么对云计算非常重要?
如何保证Linux服务器的安全性?关键策略大揭秘!
技术干货
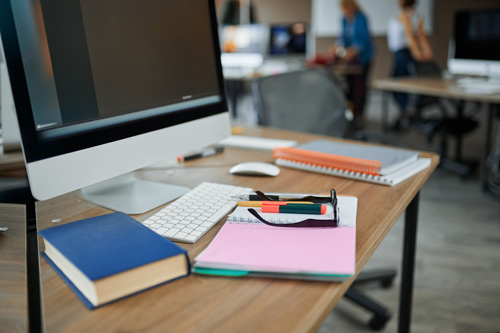
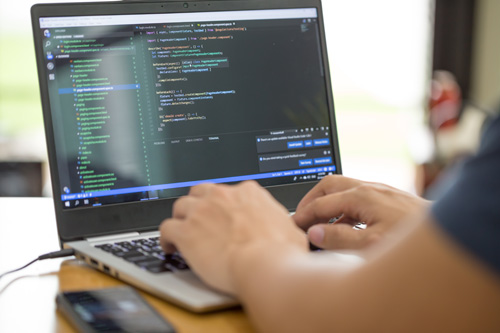
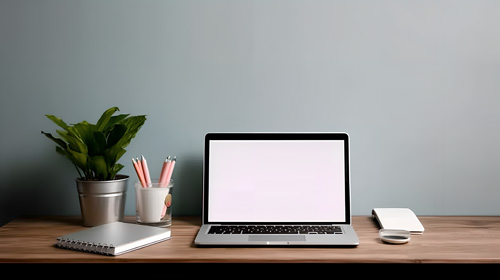
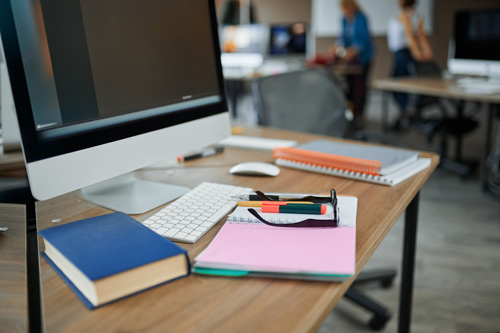
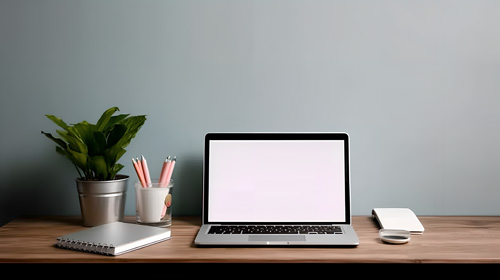
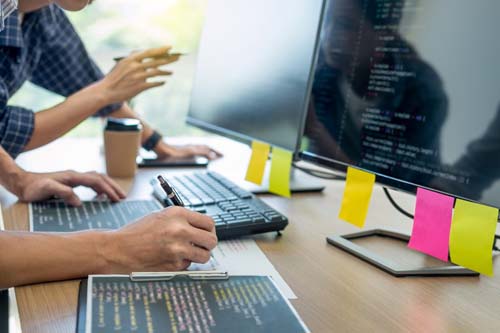
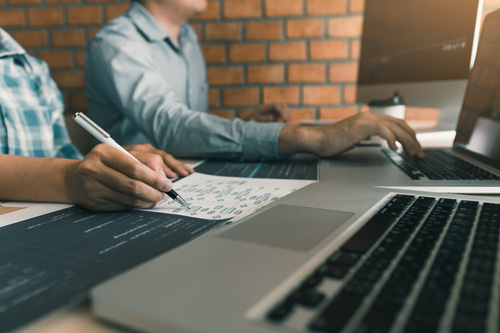